2D Finite Element Analysis of Strut Using MATLAB: A Comprehensive Guide
May 23, 2023
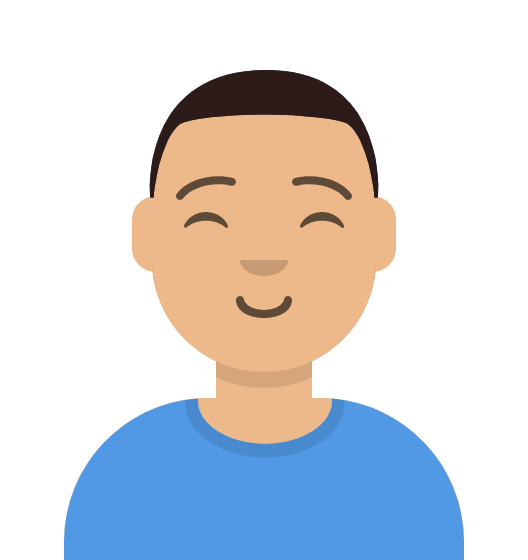
Dr. James Smith
United States
MATLAB
Dr. James Smith, a seasoned mechanical engineer and MATLAB specialist, brings over 10 years of experience in finite element analysis. Dr. Smith provides a comprehensive understanding of 2D finite element analysis of struts.
Need assistance on Assignments on 2D finite element analysis of struts using MATLAB? Look no further. As an expert in structural analysis and MATLAB programming, I offer personalized guidance and support. Whether you have specific questions, need help with implementation, or want to dive deeper into the subject, I'm here to assist you in your journey. Let's unlock the potential of MATLAB in strut analysis together.
Engineering professionals use the powerful numerical technique known as finite element analysis (FEA) to simulate and examine how structures behave under various loading scenarios. By examining the behavior of a basic strut using MATLAB, we will explore the idea of 2D finite element analysis in this blog post. The intention is to offer a thorough, step-by-step tutorial that explains the theory behind FEA and illustrates how it can be used in practice using MATLAB code.
Engineering professionals use the powerful numerical technique known as finite element analysis (FEA) to simulate and examine how structures behave under various loading scenarios. By examining the behavior of a basic strut using MATLAB, we will explore the idea of 2D finite element analysis in this blog post. The intention is to offer a thorough, step-by-step tutorial that explains the theory behind FEA and illustrates how it can be used in practice using MATLAB code.
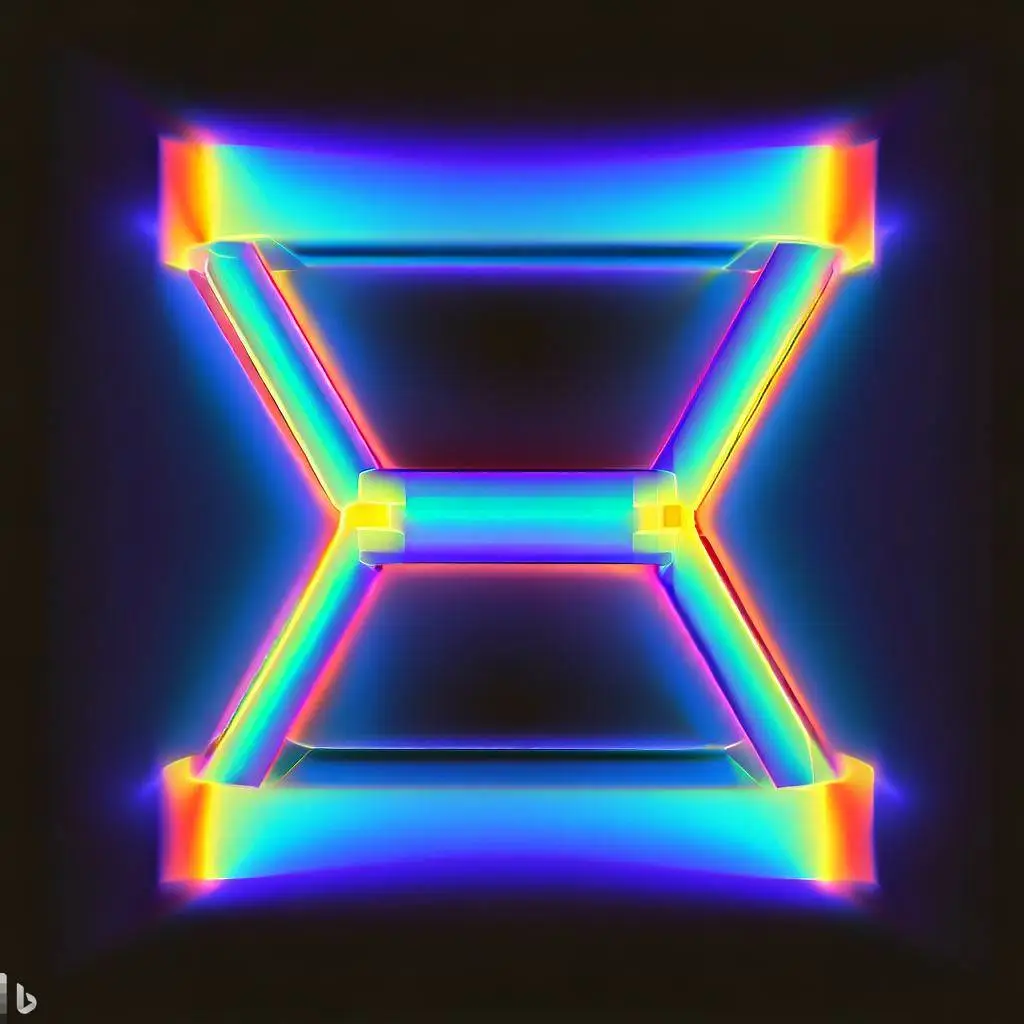
Strut Analysis:
A strut is a structural element used in engineering applications to offer support and stability. We can examine the strut's behavior and ascertain its response by putting it under various loads. Finite element analysis, which is based on breaking the structure into small elements and approximating its behavior using mathematical models, can be used to perform this analysis.
Fundamentals of the Finite Element Method (FEM):
A numerical method for solving partial differential equations and examining the behavior of complex systems is the finite element method. FEM separates the structure into more compact areas or elements, which are connected at particular locations known as nodes. We can ascertain the system's overall behavior by resolving the equations for each component.
The strut must be discretized into smaller elements in order to conduct a finite element analysis. In this illustration, we'll take a 2D strut and separate it into three triangular components. Each element has a unique shape function that represents the behavior within it, and each element is defined by its nodes.
Element Types and Shape Functions:
Depending on the geometry and complexity of the structure, different element types can be used in FEA. We'll use linear triangular elements throughout this blog. A straightforward bilinear function that roughly approximates the displacement inside the element serves as the shape function for a linear triangular element.
Construction of the Global Stiffness Matrix:
A global stiffness matrix can be used to describe the behavior of the entire structure. By combining the stiffness contributions from each element, the global stiffness matrix is created. The relationship between the applied forces and the resulting displacements is represented by the stiffness matrix.
Boundary Conditions and Applied Loads:
We must apply boundary conditions and loads to the strut model in order to simulate real-world scenarios. Loads are a representation of the external forces acting on the structure, while boundary conditions specify the constraints placed on it. We can precisely simulate the behavior of the strut under particular conditions by including these conditions.
After applying the boundary conditions and loads and assembling the global stiffness matrix, we can solve the system of equations to determine the displacements and stresses inside the structure. Numerous numerical solvers are available in MATLAB and can be used to quickly resolve complicated equation systems.
Results post-processing:
After obtaining the displacements and stresses, we can further process the data to draw out important details. This may entail calculating maximum displacements or stresses, visualizing the deformed shape of the strut, and assessing additional performance metrics.
Implementation of MATLAB:
Let's now examine how the 2D finite element analysis of the strut was implemented in MATLAB. The step-by-step process covered in the earlier sections is illustrated in the code provided below.
% MATLAB code for 2D Finite Element Analysis of a Strut
% Step 1: Define material properties and dimensions
E = 210e9; % Young's modulus (Pa)
A = 0.01; % Cross-sectional area (m^2)
L = 1; % Length of the strut (m)
% Step 2: Define the number of elements and nodes
numElements = 10;
numNodes = numElements + 1;
% Step 3: Generate the node coordinates
x = linspace(0, L, numNodes);
y = zeros(1, numNodes);
% Step 4: Generate the element connectivity matrix
elements = [1:numElements; 2:numNodes-1; 3:numNodes];
% Step 5: Assemble the global stiffness matrix
K = zeros(numNodes);
for i = 1:numElements
elementNodes = elements(:, i);
x1 = x(elementNodes(1));
x2 = x(elementNodes(2));
y1 = y(elementNodes(1));
y2 = y(elementNodes(2));
L = sqrt((x2-x1)^2 + (y2-y1)^2);
k = (E*A/L) * [1 -1; -1 1];
K(elementNodes, elementNodes) = K(elementNodes, elementNodes) + k;
end
% Step 6: Apply boundary conditions and loads
% (Note: This step will depend on the specific problem and constraints)
% Step 7: Solve the system of equations
% (Note: This step will depend on the specific problem and solver)
% Step 8: Post-process the results
% (Note: This step will depend on the specific problem and desired output)
% Step 9: Visualize the results
% (Note: This step will depend on the specific problem and desired output)
Let's dissect the MATLAB code step by step and explain each one in more detail:
% MATLAB code for 2D Finite Element Analysis of a Strut
% Step 1: Define material properties and dimensions
E = 210e9; % Young's modulus (Pa)
A = 0.01; % Cross-sectional area (m^2)
L = 1; % Length of the strut (m)
We specify the strut's dimensions and material characteristics in this step. E stands for the Young's modulus, A for the cross-sectional area, and L for the strut length.
% Step 2: Define the number of elements and nodes
numElements = 10;
numNodes = numElements + 1;
Here, we define the strut's element and node counts. There will be 11 nodes (including the endpoints) in this example because we're assuming there are 10 elements.
% Step 3: Generate the node coordinates
x = linspace(0, L, numNodes);
y = zeros(1, numNodes);
The node coordinates are generated in this step. The linspace function, which produces evenly spaced points between 0 and L, is used to create the array "x." We set the 'y' array's initial value to zeros because it is assumed that the strut is in a 2D plane.
% Step 4: Generate the element connectivity matrix
elements = [1:numElements; 2:numNodes-1; 3:numNodes];
The element connectivity matrix is created here. Each element's connectivity between nodes is described. The matrix 'elements' is a 3xnumElements matrix, where each column denotes an element and the corresponding node numbers are represented by the row values.
% Step 5: Assemble the global stiffness matrix
K = zeros(numNodes);
for i = 1:numElements
elementNodes = elements(:, i);
x1 = x(elementNodes(1));
x2 = x(elementNodes(2));
y1 = y(elementNodes(1));
y2 = y(elementNodes(2));
L = sqrt((x2-x1)^2 + (y2-y1)^2);
k = (E*A/L) * [1 -1; -1 1];
K(elementNodes, elementNodes) = K(elementNodes, elementNodes) + k;
End
This step entails putting together the global stiffness matrix "K." The relationship between the applied forces and the resulting displacements is represented by the stiffness matrix. We set up 'K' as a zero-filled matrix. The element stiffness matrix (k) for each element is then determined based on the element's dimensions and the material's properties. Based on the connectivity of the element, the global stiffness matrix 'K' is increased by the element stiffness matrix at the corresponding locations.
% Step 6: Apply boundary conditions and loads
% (Note: This step will depend on the specific problem and constraints)
As you would apply the particular boundary conditions and loads in accordance with your problem, step 6 is left as a comment. Loads are a representation of the external forces acting on the structure, while boundary conditions specify the constraints placed on it.
% Step 7: Solve the system of equations
% (Note: This step will depend on the specific problem and solver)
The comment at step 7 explains that the method you use to solve the system of equations will depend on the particular issue at hand. Numerous numerical solvers are available in MATLAB and can be used to quickly resolve complicated equation systems.
% Step 8: Post-process the results
% (Note: This step will depend on the specific problem and desired output)
Similar to Step 7, Step 8 is a comment because how the results are post-processed depends on the particular issue and the desired result. It might entail calculating maximum displacements or stresses, visualizing the strut's deformed shape, and assessing additional performance metrics.
% Step 9: Visualize the results
% (Note: This step will depend on the specific problem and desired output)
Step 9 is also a comment, indicating that how the results are visualized depends on the particular issue at hand and the desired outcome. Plotting the deformed shape, stress distribution, or any other pertinent visualization may be necessary.
% End of the MATLAB code
The provided MATLAB code ends with this line, merely.
Conclution:
Engineers can simulate and examine the behavior of complex structures using the flexible tool known as finite element analysis. By examining the behavior of a strut, we examined the idea of 2D finite element analysis in this blog. The strut's response to various loading conditions could be better understood by breaking it up into smaller elements and using FEA principles. The provided MATLAB code can be expanded and altered to suit particular engineering problems, and it serves as a starting point for performing 2D FEA on a variety of structures.
This blog only touches on the vast field of finite element analysis. Studying various element types, accounting for nonlinear material behavior, and examining more intricate structures are all possible avenues for further investigation. Engineers can gain important insights into the behavior of structures and make wise design decisions by mastering the FEA principles and how MATLAB implements them.